How To Change The Color Of An Object With A Mouse Click Java
A JComboBox
, which lets the user cull i of several choices, can have 2 very different forms. The default form is the uneditable philharmonic box, which features a push button and a drop-down list of values. The second class, chosen the editable combo box, features a text field with a small button abutting information technology. The user tin can type a value in the text field or click the button to brandish a driblet-downwards list. Hither's what the two forms of combo boxes look like in the Java await and feel:
Combo boxes require niggling screen space, and their editable (text field) form is useful for letting the user quickly choose a value without limiting the user to the displayed values. Other components that can display ane-of-many choices are groups of radio buttons and lists. Groups of radio buttons are generally the easiest for users to understand, just philharmonic boxes can be more than appropriate when infinite is limited or more than a few choices are available. Lists are not terribly attractive, merely they're more appropriate than combo boxes when the number of items is large (say, over 20) or when selecting multiple items might be valid.
Because editable and uneditable combo boxes are then unlike, this department treats them separately. This section covers these topics:
- Using an Uneditable Combo Box
- Handling Events on a Combo Box
- Using an Editable Combo Box
- Providing a Custom Renderer
- The Philharmonic Box API
- Examples that Use Combo Boxes
Using an Uneditable Philharmonic Box
The awarding shown here uses an uneditable combo box for choosing a pet picture:

The following lawmaking, taken from ComboBoxDemo.java
, creates an uneditable combo box and sets it upward:
String[] petStrings = { "Bird", "Cat", "Dog", "Rabbit", "Hog" }; //Create the philharmonic box, select item at alphabetize iv. //Indices start at 0, so four specifies the pig. JComboBox petList = new JComboBox(petStrings); petList.setSelectedIndex(4); petList.addActionListener(this);
This combo box contains an array of strings, only you could just every bit hands use icons instead. To put anything else into a combo box or to customize how the items in a combo box look, you demand to write a custom renderer. An editable philharmonic box would as well demand a custom editor. Refer to Providing a Custom Renderer for data and an instance.
The preceding code registers an action listener on the combo box. To see the action listener implementation and learn almost other types of listeners supported by philharmonic box, refer to Handling Events on a Combo Box.
No thing which constructor yous use, a combo box uses a combo box model to comprise and manage the items in its card. When you initialize a philharmonic box with an array or a vector, the combo box creates a default model object for you lot. As with other Swing components, you lot can customize a philharmonic box in office by implementing a custom model — an object that implements the ComboBoxModel
interface.
Annotation:
Be careful when implementing a custom model for a philharmonic box. The JComboBox
methods that change the items in the combo box'south card, such equally insertItemAt
, piece of work only if the data model implements the MutableComboBoxModel
interface (a subinterface of ComboBoxModel
). Refer to the API tables to encounter which methods are affected.
Something else to watch out for — fifty-fifty for uneditable combo boxes — is ensuring that your custom model fires list information events when the philharmonic box'due south data or state changes. Even immutable combo box models, whose data never changes, must burn down a listing data event (a CONTENTS_CHANGED
event) when the selection changes. One fashion to go the list data event firing code for gratis is to make your combo box model a subclass of AbstractListModel
.
Handling Events on a Combo Box
Hither's the lawmaking from ComboBoxDemo.java
that registers and implements an action listener on the combo box:
public class ComboBoxDemo ... implements ActionListener { . . . petList.addActionListener(this) { . . . public void actionPerformed(ActionEvent due east) { JComboBox cb = (JComboBox)e.getSource(); Cord petName = (String)cb.getSelectedItem(); updateLabel(petName); } . . . }
This action listener gets the newly selected particular from the philharmonic box, uses it to compute the proper noun of an image file, and updates a label to brandish the image. The combo box fires an action upshot when the user selects an item from the combo box's menu. See How to Write an Activity Listener, for full general information about implementing action listeners.
Combo boxes likewise generate detail events, which are fired when any of the items' selection state changes. Only i detail at a fourth dimension can be selected in a combo box, so when the user makes a new pick the previously selected item becomes unselected. Thus two item events are fired each time the user selects a different detail from the carte du jour. If the user chooses the same item, no item events are fired. Use addItemListener
to register an item listener on a combo box. How to Write an Particular Listener gives general information about implementing item listeners.
Although JComboBox
inherits methods to annals listeners for low-level events — focus, key, and mouse events, for example — nosotros recommend that you lot don't listen for depression-level events on a combo box. Here's why: A combo box is a compound component — it is comprised of two or more other components. The combo box itself fires loftier-level events such equally activity events. Its subcomponents burn low-level events such as mouse, key, and focus events. The low-level events and the subcomponent that fires them are look-and-feel-dependent. To avoid writing wait-and-feel-dependent code, you should listen just for high-level events on a compound component such as a philharmonic box. For information nigh events, including a word virtually loftier- and low-level events, refer to Writing Effect Listeners.
Using an Editable Combo Box
Here's a picture of a demo awarding that uses an editable combo box to enter a pattern with which to format dates.
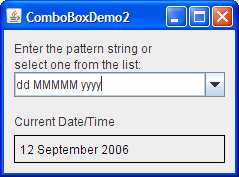
Try this:
- Click the Launch button to run the ComboBox2 Demo using Java™ Web Start (download JDK 7 or afterward). Alternatively, to compile and run the instance yourself, consult the example index.
- Enter a new blueprint by choosing one from the combo box'south carte. The programme reformats the current date and time.
- Enter a new pattern by typing ane in and pressing Enter. Again the plan reformats the current date and time.
The post-obit code, taken from ComboBoxDemo2.java
, creates and sets upward the combo box:
String[] patternExamples = { "dd MMMMM yyyy", "dd.MM.yy", "MM/dd/yy", "yyyy.MM.dd Thousand 'at' hh:mm:ss z", "EEE, MMM d, ''yy", "h:mm a", "H:mm:ss:SSS", "Thousand:mm a,z", "yyyy.MMMMM.dd GGG hh:mm aaa" }; . . . JComboBox patternList = new JComboBox(patternExamples); patternList.setEditable(true); patternList.addActionListener(this);
This lawmaking is very similar to the previous example, but warrants a few words of explanation. The assuming line of code explicitly turns on editing to allow the user to type values in. This is necessary considering, past default, a philharmonic box is not editable. This particular instance allows editing on the philharmonic box because its menu does not provide all possible date formatting patterns, just shortcuts to oft used patterns.
An editable combo box fires an activeness event when the user chooses an item from the menu and when the user types Enter. Notation that the menu remains unchanged when the user enters a value into the combo box. If you want, you tin can hands write an activity listener that adds a new particular to the combo box's menu each time the user types in a unique value.
See Internationalization to learn more about formatting dates and other types of data.
Providing a Custom Renderer
A combo box uses a renderer to brandish each item in its carte du jour. If the combo box is uneditable, it also uses the renderer to display the currently selected item. An editable combo box, on the other hand, uses an editor to display the selected item. A renderer for a combo box must implement the ListCellRenderer
interface. A combo box's editor must implement ComboBoxEditor
. This section shows how to provide a custom renderer for an uneditable combo box.
The default renderer knows how to return strings and icons. If y'all put other objects in a combo box, the default renderer calls the toString
method to provide a string to brandish. You can customize the style a combo box renders itself and its items by implementing your own ListCellRenderer
.
Here's a movie of an application that uses a combo box with a custom renderer:

Click the Launch button to run the CustomComboBox Demo using Java™ Web Commencement (download JDK 7 or later). Alternatively, to compile and run the case yourself, consult the case index.
The full source code for this case is in CustomComboBoxDemo.java
. To get the image files it requires, consult the example index.
The following statements from the example create an instance of ComboBoxRenderer
(a custom class) and ready the example equally the philharmonic box's renderer:
JComboBox petList = new JComboBox(intArray); . . . ComboBoxRenderer renderer = new ComboBoxRenderer(); renderer.setPreferredSize(new Dimension(200, 130)); petList.setRenderer(renderer); petList.setMaximumRowCount(three);
The last line sets the combo box's maximum row count, which determines the number of items visible when the card is displayed. If the number of items in the combo box is larger than its maximum row count, the menu has a scroll bar. The icons are pretty big for a menu, and so our lawmaking limits the number of rows to 3. Hither'south the implementation of ComboBoxRenderer
, a renderer that puts an icon and text side-past-side:
class ComboBoxRenderer extends JLabel implements ListCellRenderer { . . . public ComboBoxRenderer() { setOpaque(true); setHorizontalAlignment(Centre); setVerticalAlignment(Heart); } /* * This method finds the epitome and text corresponding * to the selected value and returns the label, set upwards * to display the text and image. */ public Component getListCellRendererComponent( JList list, Object value, int index, boolean isSelected, boolean cellHasFocus) { //Become the selected index. (The index parameter isn't //e'er valid, so simply use the value.) int selectedIndex = ((Integer)value).intValue(); if (isSelected) { setBackground(list.getSelectionBackground()); setForeground(list.getSelectionForeground()); } else { setBackground(list.getBackground()); setForeground(list.getForeground()); } //Set the icon and text. If icon was null, say so. ImageIcon icon = images[selectedIndex]; String pet = petStrings[selectedIndex]; setIcon(icon); if (icon != zilch) { setText(pet); setFont(list.getFont()); } else { setUhOhText(pet + " (no paradigm available)", list.getFont()); } return this; } . . . }
As a ListCellRenderer
, ComboBoxRenderer
implements a method called getListCellRendererComponent
, which returns a component whose paintComponent
method is used to display the philharmonic box and each of its items. The easiest way to display an image and an icon is to use a characterization. And so ComboBoxRenderer
is a subclass of label and returns itself. The implementation of getListCellRendererComponent
configures the renderer to brandish the currently selected icon and its description.
These arguments are passed to getListCellRendererComponent
:
-
JList list
— a listing object used backside the scenes to brandish the items. The example uses this object's colors to gear up up foreground and background colors. -
Object value
— the object to render. AnInteger
in this instance. -
int index
— the index of the object to render. -
boolean isSelected
— indicates whether the object to render is selected. Used past the example to make up one's mind which colors to employ. -
boolean cellHasFocus
— indicates whether the object to render has the focus.
Note that combo boxes and lists utilise the same type of renderer — ListCellRenderer
. You tin save yourself some time past sharing renderers between combo boxes and lists, if it makes sense for your program.
The Philharmonic Box API
The following tables listing the usually used JComboBox
constructors and methods. Other methods you are about likely to invoke on a JComboBox
object are those it inherits from its superclasses, such every bit setPreferredSize
. Come across The JComponent API for tables of normally used inherited methods.
The API for using combo boxes falls into two categories:
- Setting or Getting the Items in the Combo Box's Menu
- Customizing the Combo Box's Functioning
Method | Purpose |
---|---|
JComboBox() JComboBox(ComboBoxModel) JComboBox(Object[]) JComboBox(Vector) | Create a combo box with the specified items in its menu. A combo box created with the default constructor has no items in the menu initially. Each of the other constructors initializes the menu from its argument: a model object, an array of objects, or a Vector of objects. |
void addItem(Object) void insertItemAt(Object, int) | Add or insert the specified object into the combo box'southward carte. The insert method places the specified object at the specified index, thus inserting information technology earlier the object currently at that index. These methods require that the combo box's data model be an instance of MutableComboBoxModel . |
Object getItemAt(int) Object getSelectedItem() | Get an item from the combo box's menu. |
void removeAllItems() void removeItemAt(int) void removeItem(Object) | Remove one or more items from the combo box's menu. These methods require that the combo box's information model exist an case of MutableComboBoxModel . |
int getItemCount() | Get the number of items in the combo box's menu. |
void setModel(ComboBoxModel) ComboBoxModel getModel() | Set or get the information model that provides the items in the philharmonic box's carte du jour. |
void setAction(Action) Action getAction() | Set or become the Action associated with the combo box. For further data, see How to Utilize Actions. |
Method or Constructor | Purpose |
---|---|
void addActionListener(ActionListener) | Add an activeness listener to the philharmonic box. The listener's actionPerformed method is called when the user selects an detail from the philharmonic box's carte du jour or, in an editable combo box, when the user presses Enter. |
void addItemListener(ItemListener) | Add together an detail listener to the combo box. The listener's itemStateChanged method is chosen when the selection state of any of the philharmonic box'due south items change. |
void setEditable(boolean) boolean isEditable() | Set or get whether the user can blazon in the combo box. |
void setRenderer(ListCellRenderer) ListCellRenderer getRenderer() | Set or become the object responsible for painting the selected item in the combo box. The renderer is used only when the philharmonic box is uneditable. If the combo box is editable, the editor is used to pigment the selected item instead. |
void setEditor(ComboBoxEditor) ComboBoxEditor getEditor() | Set or become the object responsible for painting and editing the selected particular in the combo box. The editor is used but when the combo box is editable. If the combo box is uneditable, the renderer is used to paint the selected item instead. |
Examples that Use Combo Boxes
This table shows the examples that use JComboBox
and where those examples are described.
Example | Where Described | Notes |
---|---|---|
ComboBoxDemo | This department | Uses an uneditable combo box. |
ComboBoxDemo2 | This department | Uses an editable combo box. |
CustomComboBoxDemo | This department | Provides a custom renderer for a combo box. |
TableRenderDemo | How to Employ Tables (Using a Philharmonic Box every bit an Editor) | Shows how to use a combo box every bit a table cell editor. |
Source: https://docs.oracle.com/javase/tutorial/uiswing/components/combobox.html
Posted by: briggspretrusiona.blogspot.com
0 Response to "How To Change The Color Of An Object With A Mouse Click Java"
Post a Comment